GSM Interfacing with MSP430
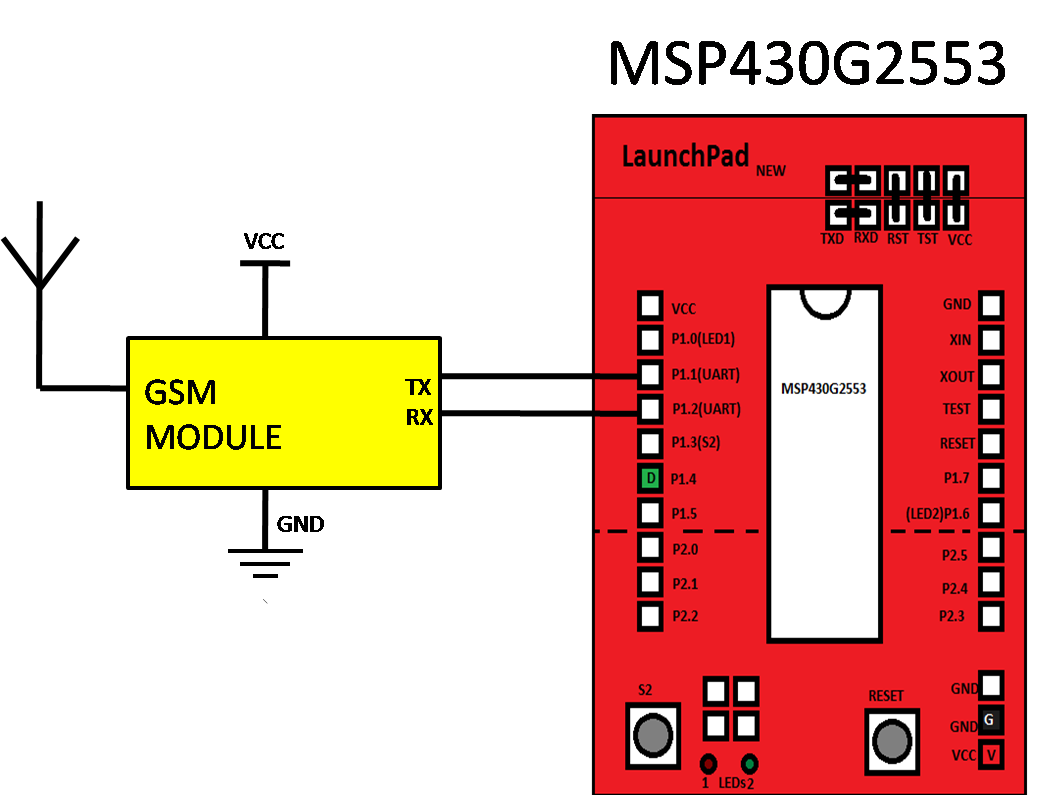
/* create file named called gsm.c and paste below given program
/*
* Project name:
GSM
* Copyright
(c) Researchdesignlab.com
* Test configuration:
MCU: MSP430G2553
Dev.Board: TI LAUNCHPAD
Oscillator: 16 MHz
Software: CCS STUDIO V.5
*/
#include "msp430g2553.h"
#include "uart.h" // ATTACH THE UART FILE WITH THE MAIN CODE
void gsm();//FUNCTION PROTOTYPE
int main(void)
{
WDTCTL = WDTPW + WDTHOLD;// STOP WATCHDOG TIMER
BCSCTL1 = CALBC1_8MHZ;// MAKE THE FREQUNCY AS PER THE LAUNCHPAD 8MHZ
DCOCTL = CALDCO_8MHZ;
uart_init(); // CALL THE UART INIT FUNCTION WHICH IS AVAILAIBLE IN THE FILE
__enable_interrupt();// ENABLE INTERRUPT
__delay_cycles(100000);
gsm();// CALL THE GSM FUNCTION
}
void gsm()
{
uart_puts((char *)"AT"); // COMMAND FOR INITIALIZING GSM
uart_putc(0x0A);//ENTER
uart_putc(0x0D);//CARRIAGE RETURN
__delay_cycles(10000000);//DELAY...WAIT FOR OK FROM GSM
uart_puts((char *)"AT+CMGF=1");//COMMUNICATION
uart_putc(0x0A);
uart_putc(0x0D);
__delay_cycles(10000000);//WAIT FOR OK
uart_puts((char *)"AT+CMGS=\"8123902188\"");//SEND A MESSAGE TO PARTICULAR NUMBER
uart_putc(0x0A);
uart_putc(0x0D);
uart_puts((char *)"hello");//SEND HELLO
uart_putc(0x1A);//CTRL Z
//AFTER HARDWARE CONFIGURATION THE MESSAGE WILL GET SEND
//ATTACH THE UART FILES OR WRITE THE CODE FOR INIT AND SENDING MESSAGE IN THE SAME FILE...
}
// end of gsm.c file
//………………………………………………………………………………………………………………………………………………………
/* create file named called urat.c and paste below given program
/*
*This is a uart.c file of the UART Hardware demo using USCI on the MSP430G2553 microcontroller.
*Set your baud rate in your terminal to 9600 8N1.
*When using your TI MSP-430 LaunchPad you will need to cross the TXD and RXD jumpers.
*/
#include "msp430g2553.h"
#include "uart.h"
#define LED BIT0
#define RXD BIT1
#define TXD BIT2
volatile unsigned int tx_flag; //Mailbox Flag for the tx_char.
volatile unsigned char tx_char; //This char is the most current char to go into the UART
volatile unsigned int rx_flag; //Mailbox Flag for the rx_char.
volatile unsigned char rx_char; //This char is the most current char to come out of the UART
/*uart_init
* Sets up the UART interface via USCI
* INPUT: None
* RETURN: None
*/
void uart_init(void)
{
P1SEL = RXD + TXD; //Setup the I/O
P1SEL2 = RXD + TXD;
P1DIR |= LED; //P1.0 red LED. Toggle when char received.
P1OUT |= LED; //LED off
UCA0CTL1 |= UCSSEL_2; //SMCLK
//8,000,000Hz, 9600Baud, UCBRx=52, UCBRSx=0, UCBRFx=1
UCA0BR0 = 52; //8MHz, OSC16, 9600
UCA0BR1 = 0; //((8MHz/9600)/16) = 52.08333
UCA0MCTL = 0x10|UCOS16; //UCBRFx=1,UCBRSx=0, UCOS16=1
UCA0CTL1 &= ~UCSWRST; //USCI state machine
IE2 |= UCA0RXIE; //Enable USCI_A0 RX interrupt
rx_flag = 0; //Set rx_flag to 0
tx_flag = 0; //Set tx_flag to 0
return;
}
/*uart_getc
* Get a char from the UART. Waits till it gets one
* INPUT: None
* RETURN: Char from UART
*/
unsigned char uart_getc() //Waits for a valid char from the UART
{
while (rx_flag == 0); //Wait for rx_flag to be set
rx_flag = 0; //ACK rx_flag
return rx_char;
}
/*uart_gets
* Get a string of known length from the UART. Strings terminate when enter is pressed or string buffer fills
* Will return when all the chars are received or a carriage return (\r) is received. Waits for the data.
* INPUT: Array pointer and length
* RETURN: None
*/
void uart_gets(char* Array, int length)
{
unsigned int i = 0;
while((i < length)) //Grab data till the array fills
{
Array[i] = uart_getc();
if(Array[i] == '\r') //If we receive a \r the master wants to end
{
for( ; i < length ; i++) //fill the rest of the string with \0 nul. Overwrites the \r with \0
{
Array[i] = '\0';
}
break;
}
i++;
}
return;
}
/*uart_putc
* Sends a char to the UART. Will wait if the UART is busy
* INPUT: Char to send
* RETURN: None
*/
void uart_putc(unsigned char c)
{
tx_char = c; //Put the char into the tx_char
IE2 |= UCA0TXIE; //Enable USCI_A0 TX interrupt
while(tx_flag == 1); //Have to wait for the TX buffer
tx_flag = 1; //Reset the tx_flag
return;
}
/*uart_puts
* Sends a string to the UART. Will wait if the UART is busy
* INPUT: Pointer to String to send
* RETURN: None
*/
void uart_puts(char *str) //Sends a String to the UART.
{
while(*str) uart_putc(*str++); //Advance though string till end
return;
}
#pragma vector = USCIAB0TX_VECTOR //UART TX USCI Interrupt
__interrupt void USCI0TX_ISR(void)
{
UCA0TXBUF = tx_char; //Copy char to the TX Buffer
tx_flag = 0; //ACK the tx_flag
IE2 &= ~UCA0TXIE; //Turn off the interrupt to save CPU
}
#pragma vector = USCIAB0RX_VECTOR //UART RX USCI Interrupt. This triggers when the USCI receives a char.
__interrupt void USCI0RX_ISR(void)
{
rx_char = UCA0RXBUF; //Copy from RX buffer, in doing so we ACK the interrupt as well
rx_flag = 1; //Set the rx_flag to 1
P1OUT ^= LED; //Notify that we received a char by toggling LED
}
// end of urat.c file
//………………………………………………………………………………………………………………………………………………………
/* create file named called urat.h and paste below given program
/*
*This is a uart.h file of the UART Hardware demo using USCI on the MSP430G2553 microcontroller.
*Set your baud rate in your terminal to 9600 8N1.
*When using your TI MSP-430 LaunchPad you will need to cross the TXD and RXD jumpers.
*/
/*rx_flag
* This flag is to be used by other modules to check and see if a new transmission has happened.
* This is READ ONLY. Do not write to it or the UART may crash.
*/
extern volatile unsigned int rx_flag;
/*uart_init
* Sets up the UART interface via USCI
* INPUT: None
* RETURN: None
*/
void uart_init(void);
/*uart_getc
* Get a char from the UART. Waits till it gets one
* INPUT: None
* RETURN: Char from UART
*/
unsigned char uart_getc();
/*uart_gets
* Get a string of known length from the UART. Strings terminate when enter is pressed or string buffer fills
* Will return when all the chars are received or a carriage return (\r) is received. Waits for the data.
* INPUT: Array pointer and length
* RETURN: None
*/
void uart_gets();
/*uart_putc
* Sends a char to the UART. Will wait if the UART is busy
* INPUT: Char to send
* RETURN: None
*/
void uart_putc(unsigned char c);
/*uart_puts
* Sends a string to the UART. Will wait if the UART is busy
* INPUT: Pointer to String to send
* RETURN: None
*/
void uart_puts(char *str);
// end of urat.h file
//………………………………………………………………………………………………………………………………………………………